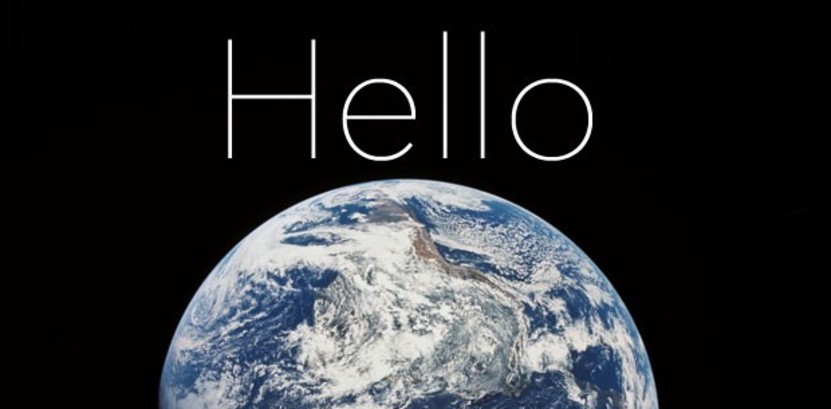
Step-by-step “Hello World” examples in Ruby, SQL, and Ruby on Rails
Continuing forward from Part 1 of this 3-part “Hello World” mini-series within our web and mobile development tutorials, here we'll walk through Ruby, Standard Query Language (SQL), and Ruby on Rails. If you have not yet completed steps 1–7 from Part 1, click here to go back and do so now. If you have, great, let's continue.
[Author's note: I wrote the first couple dozen tutorials in this series a few years ago and I'm in the process of updating the content to reflect the evolution of best practices in the industry. Please comment if you see anything I missed that should be updated. Thanks!]
Step 8: “Hello World” in Ruby
Here let's jump back into our terminal and use a neat tool called Interactive Ruby (IRB):
~/apps/OurAgendaApp $ irb 1.9.3p194 :001 > a = "Hello World" => "Hello World" 1.9.3p194 :003 > a => "Hello World"
Sweet. That was easy. Enter “exit” to get out of IRB.
In our session there we loaded the string “Hello World” into memory via the variable a, which we could then do interesting things with … like what we did on the second line to return what a contained :)
Step 9: “Hello World” in SQL
Let's jump into a session of PostgreSQL's command line interface:
~/apps/OurAgendaApp $ psql -d OurAgendaApp_development psql (9.1.2) Type "help" for help. OurAgendaApp_development=# CREATE TABLE users ( OurAgendaApp_development(# id SERIAL, OurAgendaApp_development(# first_name varchar(255), OurAgendaApp_development(# last_name varchar(255) OurAgendaApp_development(# ); NOTICE: CREATE TABLE will create implicit sequence "users_id_seq" for serial column "users.id" CREATE TABLE OurAgendaApp_development=# INSERT INTO users (first_name, last_name) VALUES ('Hello', 'World'); INSERT 0 1 OurAgendaApp_development=# SELECT * FROM users; id | first_name | last_name ----+------------+----------- 1 | Hello | World (1 row) OurAgendaApp_development=# DROP TABLE users; DROP TABLE OurAgendaApp_development=# \q ~/apps/OurAgendaApp $
Here we got into our psql session and created a users table, inserted the first and last names, and selected them for display to get our Hello World. We deleted the users table at the end because we're going to have Rails do the magic for us next. In fact, we may never come back to the psql session again, as part of the greatness of Rails is that it will handle all the SQL for you, but we felt it was important for you to at least get a small idea of what Rails is going to be doing for you a bunch under the hood.
Step 10: “Hello World” in Ruby on Rails
Let's first generate a model.
$ rails g model User first_name:string last_name:string
This will create your model file, your migration file, and your test files, which will get into in greater depth later. For now, take a quick peek at the generated migration file in your “#{Rails.root}/db/migrate” folder, it should look like this:
class CreateUsers < ActiveRecord::Migration def change create_table :users do |t| t.string :first_name t.string :last_name t.timestamps end end end
Pretty neat. This created a Migration class that has a method called “change” which will either create your table or drop your table, depending on how you call it (hopefully we only need to call it once, but sometimes you need to rollback changes, and Rails can make this easy with migration files). The “t.timestamps” puts in your “created_at” and “updated_at” columns which Rails will automatically stamp for you as you create and save your records. You should read a little bit about migrations here before continuing.
Done? Cool. Now let's run this migration file:
~/apps/OurAgendaApp $ rake db:migrate
That should have run successfully and told you how long it took to migrate (like 0.0043s or something).
Now let's get into our Rails console session and create a user:
~/apps/OurAgendaApp $ rails c Loading development environment (Rails 3.2.9) 1.9.3p194 :001 > User.create(first_name: "Hello", last_name: "World") . . . 1.9.3p194 :002 > exit
In place of those three dots, you should have seen the SQL that Rails ran for you to create your user, including the inserted timestamps, and you should have seen the output of the returned user object listing its attributes.
Now let's go ahead and do some Rails magic to print this in our browser. Open back up your index.html.erb file and add to the bottom:
<p id="p_HTML">Hello World</p> <p id="p_CSS"></p> <p id="p_JavaScript"> <script type="text/javascript"> document.write("Hello World"); </script> <p id="p_jQuery"></p> <script type="text/javascript"> $(document).ready(function(){ $("#p_jQuery").text("Hello World") }); </script> <p id="p_CoffeeScript"></p> <% user = User.first %> <p id="p_Rails"><%= user.first_name %> <%= user.last_name %> </p>
Now make sure your “rails s” is running in your terminal and reload your browser and you should see a sixth Hello World.
Look in the log output in your terminal and you should see how Rails ran an SQL query to pull your user from the database. It should look like this:
Started GET "/" for 127.0.0.1 at 2012-12-28 16:39:07 -0800 Connecting to database specified by database.yml Processing by HomeController#index as HTML User Load (0.6ms) SELECT "users".* FROM "users" LIMIT 1 Rendered home/index.html.erb within layouts/application (53.5ms) Completed 200 OK in 290ms (Views: 275.8ms | ActiveRecord: 13.8ms)
In general, it's always helpful to monitor the log output in development so you know what's going on under the hood, how long things take to render, how fast or slow your SQL queries take, etc…
- — -
In our next post in this AppDevIntro series we'll finish our “Hello World” trilogy by walking through Hello World examples in JSON, Eco, and Backbone.js. Previous post: Step-by-step “Hello World” examples in HTML, CSS, SASS, JavaScript, jQuery, and CoffeeScript.
Startup Rocket
We help entrepreneurs ideate, validate, create, grow, and fund new ventures.
Based on decades of startup experience, our team has developed a comprehensive operations framework, a collaborative online tool, and a personalized coaching experience to help take your business to the next level.