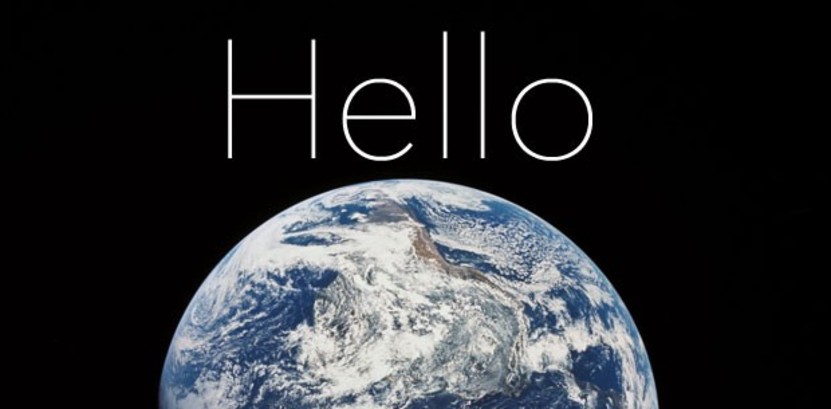
Step-by-step “Hello World” examples in HTML, CSS, SASS, JavaScript, jQuery, and CoffeeScript
We're now at the point in our web and mobile development series where we'll jump right into setting up the app we'll be building step-by-step together. This will be a practical, hands-on introduction to a dozen web-app related programming languages, frameworks, and libraries. When we're done with this and the next two posts, we'll have the foundation of our app ready to receive and merge in the pretty stuff from our visual designer (which we'll do later in this series). You ready? Let's go.
[Author's note: I wrote the first couple dozen tutorials in this series a few years ago and I'm in the process of updating the content to reflect the evolution of best practices in the industry. Please comment if you see anything I missed that should be updated. Thanks!]
Step 1: Ensure your local environment is setup for developing Ruby on Rails apps.
If you haven't done this yet, Mac users go here and Windows users go here. Setup your computer per the applicable tutorial's instructions.
Windows users will be using Git Bash heavily from here forward, Mac users will use Terminal, respectively.
Windows users take note that when we say “your Terminal” this means your Git Bash.
Step 2: Setup your new Rails app
Open your terminal and type in the following (below). Remember, enter everything after the “$” on each line. The “~” is shorthand for your personal user's home directory. When your working directory changes, this path output will change to let you know where you are at in your file system.
~ $ mkdir apps ~ $ cd apps ~/apps $ gem install bundler --no-ri --no-rdoc ~/apps $ gem install rails --no-ri --no-rdoc ~/apps $ rails new OurAgendaApp -d postgresql
Here you are creating your “Rails.root” directory (which is ~/apps/OurAgendaApp — remember this!), installing the latest bundler gem and Rails version (without installing the documentation, ‘speeds it up), and then creating your “OurAgendaApp” Rails app folder and files with an assumed Postgresql database connection (we'll get into this more below).
The Rails setup also automatically runs “bundle install” — which downloads and installs the default set of RubyGems needed to run your app.
At this point if you've run into any errors (you shouldn't, in theory, if you've followed your setup tutorial in Step 1 closely) then you'll need to follow what the error messages say and Google around to setup your local environment to be ready (let us know if you had to do this so we can help others out, too).
Step 3: “Hello World” in HTML
We're first going to create a Rails controller, its initial index view, and tell our Rails app to route requests to “/” to this controller action called “index.”
~/apps $ cd OurAgendaApp ~/apps/OurAgendaApp $ rails g controller home . . . #these dots mean it will output stuff ~/apps/OurAgendaApp $ rm public/index.html #don't need this ~/apps/OurAgendaApp $ touch app/views/home/index.html.erb
That last command created an empty file called index.html.erb, and it's full path is:
“#{Rails.root}<strong>/app/views/home/index.html.erb</strong>”
In your code editor (i.e. Sublime Text 2), open up your “#{Rails.root}” folder (remember, this is ~/apps/OurAgendaApp) and click in the directory tree to bring up your index.html.erb file.
This file should be blank. On the first line go ahead and write:
<p id="p_HTML">Hello World</p>
Save it, and then click over in your directory tree and open the
#{Rails.root}/config/routes.rb file. Around lines 49–51 you should see:
# You can have the root of your site routed with "root" # just remember to delete public/index.html. # root :to => 'welcome#index'
Go ahead and uncomment that last line and change ‘welcome' to ‘home':
# You can have the root of your site routed with "root" # just remember to delete public/index.html. <strong>root :to => 'home#index'</strong>
Save it and go back to your Terminal window and let's setup your database user (Windows users, FYI, will need to create the “OurAgendaApp” user following the general instructions noted at the bottom of the Windows setup tutorial). Mac users can just enter this…
~/apps/OurAgendaApp $ createuser --login --createdb OurAgendaApp
…and say yes to this user being a superuser.
Windows users — but not Mac users — will need to set a password for their OurAgendaApp postgresql user and enter that password on line 21 of the “#{Rails.root}/config/database.yml” file.
Ok, now you should be able to create the development database via:
~/apps/OurAgendaApp $ rake db:create
…and then fire up the Rails server via:
~/apps/OurAgendaApp $ rails s
This should dump out a few lines in your Terminal saying something about WEBrick starting on port 3000. Now you can open up your browser (use Chrome) and go to http://localhost:3000/ and you should see your Hello World from the HTML you entered into your
#{Rails.root}/app/views/home/index.html.erb file.
If you got an error, re-read the steps above and try to Google around and follow the error messages to resolve your issue. If you're still stuck, please contact us and we'll do our best to help.
Step 4: “Hello World” in CSS and SASS
First, right-click in your Hello World output screen and “view source.” It should look something like this:
This is the final HTML output from your Rails app. Take a look at your “#{Rails.root}/app/views/layouts/application.html.erb” file, which should look like this:
<!DOCTYPE html> <html> <head> <title>OurAgendaApp</title> <%= stylesheet_link_tag "application", :media => "all" %> <%= javascript_include_tag "application" %> <%= csrf_meta_tags %> </head> <body> <%= yield %> </body> </html>
What you are looking at here is Embedded Ruby inside HTML (this should look familiar from our ERB intro post). Those tag lines in the
do the magic to output what you saw above in the browser source, and the yield line embeds the content of the index.html.erb file.For our purposes in this step, we'll concern ourselves with that home.css file, which was generated from the “#{Rails.root /app/assets/stylesheets/home.css.scss” file.
First, open back up your index.html.erb file from above and add in a second line:
<p id="p_HTML">Hello World
Now open the “#{Rails.root}/app/assets/stylesheets/home.css.scss” file. Scss stands for a SASS file, which is a handy meta-level CSS language. First let's add in some CSS:
Now reload your browser and you should see two Hello World outputs. Nice!
What you did here was use CSS to set a width and height of your second paragraph element and set it's background to a PNG image (we made for you) that says Hello World.
Here's how we could write the same thing using SASS:
You can see how this can be useful if you use the same colors, widths, background images, etc… in multiple places — it helps you not repeat yourself (an important concept in programming called DRY — Don't Repeat Yourself). Sass has other powerful tools such as mixins, selector inheritance, and a clean nesting syntax…learn more about these on the Sass website.
Step 5: “Hello World” in JavaScript
Back in your index.html.erb file add in a third paragraph tag containing JavaScript like this:
<p id="p_HTML">Hello World</p> <p id="p_CSS"></p> <p id="p_JavaScript"> <script type="text/javascript"> document.write("Hello World"); </script> </p>
Now reload your browser. Boom. That was easy. We inserted a script tag and called a method called write on our document object, passing it the string “Hello World” which our browser interpreted correctly and wrote inside our third paragraph tag.
Step 6: “Hello World” in jQuery
Here we'll setup a fourth paragraph in our index.html.erb file and then add a script tag that tells the browser to fill it with a Hello World a split second after it loads:
<p id="p_HTML">Hello World</p> <p id="p_CSS"></p> <p id="p_JavaScript"> <script type="text/javascript"> document.write("Hello World"); </script> <p id="p_jQuery"></p> <script type="text/javascript"> $(document).ready(function(){ $("#p_jQuery").text("Hello World") }); </script>
As you recall from our jQuery intro post, the $() notation allows us to select objects in our browser (such as the document object, specific elements, etc…) and do things with them.
Here we called the jQuery ready() method on the document object, which is triggered when the browser has finished loading things (handy when you want to make sure everything is available for you to use).
After it's triggered then we inserted the string Hello World via the jQuery text()method into our p_jQuery paragraph.
Step 7: “Hello World” in CoffeeScript
First let's go ahead and add another paragraph tag in our index.html.erb file:
<p id="p_HTML">Hello World</p> <p id="p_CSS"></p> <p id="p_JavaScript"> <script type="text/javascript"> document.write("Hello World"); </script> <p id="p_jQuery"></p> <script type="text/javascript"> $(document).ready(function(){ $("#p_jQuery").text("Hello World") }); </script> <p id="p_CoffeeScript"></p>
Now, open up the “#{Rails.root}/app/assets/javascripts/home.js.coffee” file and add:
# Place all the behaviors and hooks # related to the matching controller here. # All this logic will automatically be available in application.js. # You can use CoffeeScript in this file: # <a href="http://jashkenas.github.com/coffee-script/">http://jashkenas.github.com/coffee-script/</a> $(document).ready -> $("#p_CoffeeScript").text("Hello World")
Now refresh your browser and you should see a fifth “Hello World” line.
More than just the neat -> thing for functions and not needing to add semicolons, CoffeeScript has a bunch of additional features that you should check out (and that we'll be using later in this series).
- — -
In our next post, we'll continue with step 8, 9, and 10, walking through “Hello World” examples with Ruby, SQL, and Rails. Previous post: Introducing the app we'll be building publicly step-by-step.
Startup Rocket
We help entrepreneurs ideate, validate, create, grow, and fund new ventures.
Based on decades of startup experience, our team has developed a comprehensive operations framework, a collaborative online tool, and a personalized coaching experience to help take your business to the next level.