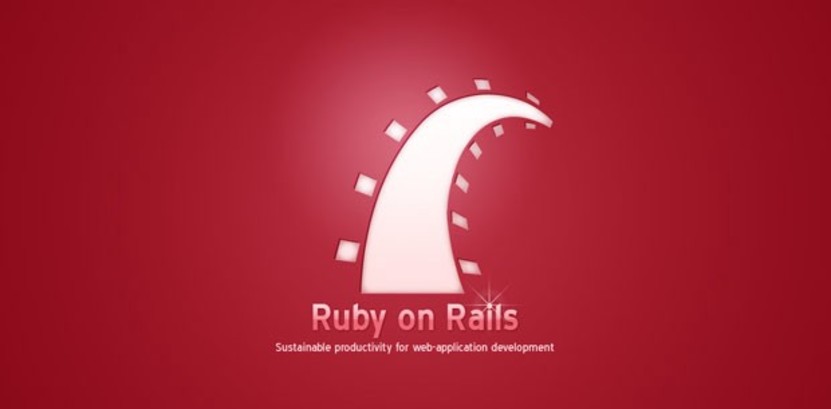
A quick introduction to Ruby on Rails
Moving forward with our tutorial series teaching beginners how to build modern-day apps for web and mobile devices, today we're going to take a brief, high-level look at Ruby on Rails and it's Model-View-Controller architecture to give you a better understanding of what all the fuss is about.
[Author's note: I wrote the first couple dozen tutorials in this series a few years ago and I'm in the process of updating the content to reflect the evolution of best practices in the industry. Please comment if you see anything I missed that should be updated. Thanks!]
When I first heard about Rails back in 2005 I didn't really “get it.” The name seemed silly. After watching the famous (now old-school) blog in 15-mins videoand diving into the code a bit, I quickly realized my PHP days were over. I committed to building every new project on this new framework, and it was one of the best decisions I've ever made (with Node.js on the scene now I've gotten more flexible, but we'll talk about that later :) ).
First, Rails is a web development framework written in Ruby. In other words, you — as the developer — write Ruby code for handling back-end things like environment setup, routing, business logic, object methods, validation, communication with a database and external services, and embedding code inside front-end documents like we talked about in the last post. I don't expect you to know what any of those things are that I just mentioned — so don't worry, we will dive into each one as we build our app step-by-step throughout this series.
Next, Rails follows a Model-View-Controller architecture. Honestly, it took me forever to figure out what this actually means, so the best way I can explain it to beginners (before we dive into just building an app of our own) is to think about an online store that has products, which I'll talk more about below.
The Model
The model is the code behind a resource (or object) of the store, such as a product. Rails has special classes called models that inherit methods fromActiveRecord::Base, which is a fancy module that serves as an Object Relational Mapper (ORM) to a relational database (like MySQL or PostgreSQL). In other words (because I'm surrrrrrrrre you followed that 100%), Rails makes it easy to create, read, update, and destroy (a common quartet often termed CRUD) objects. For example:
store = Store.new store.name = "Best Buy" store.description = "An electronics store..." store.save s = Store.find_by_name("Best Buy") s.name <em>#this outputs "Best Buy"</em> s.description = "A showroom for Amazon.com" s.save <em>#this updates our store in the database </em> s.destroy
Rails provides an easy way, using Ruby code, to setup our database table so that we don't need to write Standard Query Language (SQL) ourselves (we can, of course, but Rails does a lot of the simple things for us). Once we set things up — which we'll get into later as we build our app — we can CRUD our objects with simple methods such as those shown above.
The model is also the place where we handle validations (i.e. only save things to our database if attributes aren't blank or meet certain criteria), and where we write custom methods, scopes, and other fancy stuff we'll talk about later.
The Controller
The controller handles the code between the routes and the views, relying heavily on model methods. In other words, if you route a request like /products/4 to the products controller, which is a classic example, then inside this controller you would have a method (also called in this context an ‘action') to do something with that ID number passed in the path:
class ProductsController < ApplicationController def show @product = Product.find_by_id(params[:id]) end end
In general, you want to keep your controllers as “skinny” as possible and put code that involves products in the model. In this case, our controller has one action, called “show”, that preps a variable called @products that will be available to embed in our associated view.
The View
Finally, the view is the code that preps what will be sent to the requesting user's browser. This is where Embedded Ruby comes in and why we devoted a quick overview post to it. Essentially, following our example above, we would have a show.html.erb file in our Rails app that would look something like this:
<div class="product_show"> <span><%= @product.name %></span> <span><%= @product.description %></span> </div>
Normally, this action would be rendered inside a another .html.erb file called a layout which contains all the high-level stuff that exists on every page headers, footers, navigation bars, etc…
The MVC Game
So that's basically it — in a general sense — which I think will be helpful for you as you dive into writing code. Simply put, a request comes through the routes, into the controller, then back to the browser after reading/writing via the model and grabbing HTML or JavaScript from view files that have embedded Ruby in them. Click here to see some different MVC diagrams to get a feel for how it works. Everyone draws it slightly differently, which is why the best way to learn is in the context of building a web app (and after you've built a few, then you get a better idea).
- — -
In our next post, we'll chat about Git, a neat version control system that most Rails developers use to house (host) their code and keep track of changes. Previous post: Embedded Ruby introduction.
Startup Rocket
We help entrepreneurs ideate, validate, create, grow, and fund new ventures.
Based on decades of startup experience, our team has developed a comprehensive operations framework, a collaborative online tool, and a personalized coaching experience to help take your business to the next level.