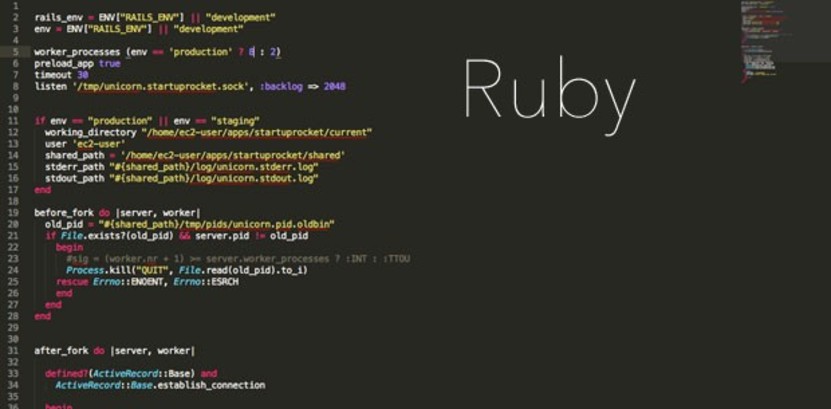
A quick introduction to Ruby
Continuing with our Introduction to web and mobile development series, we'll be making an important jump here from front-end code rendered by web browsers (e.g.HTML, CSS, JavaScript, etc.) to back-end code (e.g. Ruby, Python, PHP, Perl, etc.) which is interpreted by behind-the-scenes on servers.
[Author's note: I wrote the first couple dozen tutorials in this series a few years ago and I'm in the process of updating the content to reflect the evolution of best practices in the industry. Please comment if you see anything I missed that should be updated. Thanks!]
Ruby is a scripting language that was developed in the mid-90s as an attempt to make server-side code cleaner, easier to read, more enjoyable to write, more flexible (i.e. following conventions from a bunch of other languages), and able to get more done with less lines of code. If you've read our quick introduction to JavaScript then you are already familiar with the idea of object-oriented programing, which allows you to setup and play around with objects like Lego pieces.
For example, let's setup a quick Store, in your Terminal, type in irb and press enter to get into “interactive Ruby”, then type in class Store; attr_accessor :name; end and press enter. You should see something like this:
$ irb :001 > class Store; attr_accessor :name; end => nil
FYI, the “$” marks the start of a shell prompt (e.g. in your Terminal program) and “>” marks the start of an irb session line. Also, Ruby is cool because you could have written that line in three lines without semicolons, like this:
class Store attr_accessor :name end
Anyway, in your irb session you now have the Store object setup. Theattr_accessor method is part of ruby's meta magic for classes and helps setup “getter” and “setter” methods for our name attribute. This means you can now do something like this:
> s1 = Store.new > s2 = Store.new > s3 = Store.new > s1.name = "Autozone" > s2.name = "Seven Eleven" > s3.name = "Best Buy" > stores = [s1, s2, s3]
As you enter each line you'll see output like => #
So, now you have a variable called stores that holds an array of store objects. This kind of setup is very common as you develop apps. Normally you retrieve an array of objects from a database and then do something with it. For our stores example, we could do:
> for s in stores; puts s.name; end
Which of course prints the name of our three stores. Ruby also allows syntax like this:
> stores.each {|s| puts s}
Which does the exact same thing.
So, take a moment to play around in your irb session. Try to rename a store. Or you can add a store to your stores array like this:
s4 = Store.new; s4.name = "Walmart"; stores << s4
And off you go. You now know Ruby in a fundamental way, and we'd encourage you to follow this 20min quickstart tutorial on the main Ruby site to dive in deeper.
— —
In our next post we'll be discussing embedded Ruby, which allows you to embed lines of ruby code in other files (e.g. HTML files), which as you'll see is extremely useful when building web pages. Previous post: jQuery introduction
Startup Rocket
We help entrepreneurs ideate, validate, create, grow, and fund new ventures.
Based on decades of startup experience, our team has developed a comprehensive operations framework, a collaborative online tool, and a personalized coaching experience to help take your business to the next level.